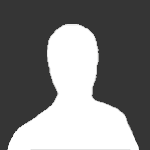
Java Strings: Best Practices for Performance
Started by
Antonher,
1 post in this topic
Create an account or sign in to comment
You need to be a member in order to leave a comment